Ever found yourself wrestling with a Java Swing JTable that simply refuses to let you edit its cells? You're not alone! The JTable, a cornerstone of Java GUI development, offers powerful tools for data display and manipulation, but unlocking its full potential, especially when it comes to cell editing, can be a surprisingly complex endeavor.
The JTable component in Java Swing, residing within the javax.swing
package, is designed to present data in a familiar tabular format. Its strength lies in its ability to act as a dynamic view of your data, offering functionalities from basic display to advanced features like sorting, filtering, and, crucially, in-place editing. However, the "out-of-the-box" behavior can sometimes be misleading, leading developers to believe editing should be enabled by default. The reality is, configuring a JTable for editing requires a deliberate approach, understanding the underlying data model, and potentially customizing editors and renderers.
Topic | Details |
---|---|
Component | JTable (Java Swing) |
Package | javax.swing.JTable |
Functionality | Displaying and manipulating tabular data. |
Key Concepts | TableModel, Cell Editors, Cell Renderers |
Additional Resources | Oracle's Java Swing Tutorial - How to Use Tables |
One common stumbling block arises from the JTable's reliance on a separate TableModel
object. The TableModel
acts as the data source for the JTable, and critically, it dictates whether or not cells are editable. A default implementation, like DefaultTableModel
, can often be used for simplicity, but even then, you must ensure its isCellEditable()
method is correctly configured. If you are using a custom TableModel
(often extending AbstractTableModel
), you have complete control over the editing behavior, but you also bear the responsibility of implementing it correctly.
- Your Guide Watch Hollywood Hindi Dubbed Movies Online 2024
- Kannada Cinemas 5 Rules A 2024 Deep Dive Updated
Consider this scenario: You've initialized a JTable, populated it with data, and embedded it within a JScrollPane for easy scrolling. You run your application, select a cell, and attempt to modify its contents, only to find that nothing happens. The cell stubbornly refuses to change. This is often a sign that the isCellEditable()
method in your TableModel
is returning false
for that particular cell (or, indeed, for all cells). The fix usually involves overriding this method to return true
based on your specific editing criteria.
Let's delve into the specifics. Imagine you're displaying employee details in a JTable, mirroring a spreadsheet-like interface. You want users to be able to directly edit the employee's salary or department but restrict changes to their employee ID. To achieve this, you would implement the isCellEditable()
method in your TableModel
as follows:
class MyTableModel extends AbstractTableModel { // ... other methods ... @Override public boolean isCellEditable(int row, int column) { // Allow editing of salary (column 2) and department (column 3) return column == 2 || column == 3; }}
In this example, only the columns representing salary and department are editable, while other columns remain read-only. This granular control is a key advantage of using a custom TableModel
.
Beyond simply enabling editing, you might also want to customize the editing component itself. By default, a JTable uses a JTextField
for editing text-based cells. However, you might need a different editor for specific data types. For instance, you could use a JComboBox
to restrict the values a user can enter in a particular column, or a JFormattedTextField
to ensure that numeric data is entered in the correct format. Swing provides a flexible mechanism for specifying custom cell editors, allowing you to tailor the editing experience to your application's specific needs.
To use a JComboBox
as an editor for a specific column (e.g., the "Sport" column in a table displaying sports preferences), you would need to create a custom TableCellEditor
. This editor would be responsible for displaying the combo box when the cell is being edited and for updating the underlying data in the TableModel
when the user selects a new value. The TableDemo
example in the Java Swing tutorial demonstrates this technique effectively.
Another common issue arises when using a JTable within a JScrollPane. If the JTable's preferred scrollable viewport width exceeds the sum of its columns' widths, you might encounter unsightly whitespace at the right edge of the table. This can be resolved by ensuring that the JTable's preferred width is correctly calculated and set, preventing the scroll pane from allocating excessive space.
Furthermore, when dealing with potentially large datasets, performance considerations become paramount. The JTable itself doesn't store or cache data; it merely acts as a view onto your data. This means that every time the JTable needs to display a cell, it queries the TableModel
for the cell's value. If the TableModel
is slow to respond (e.g., due to database access or complex calculations), the JTable's performance will suffer. Caching data within the TableModel
or using a more efficient data structure can significantly improve performance in such cases.
Consider a scenario where you're fetching data from a database to populate your JTable. Instead of directly querying the database every time the JTable requests a cell value, you could load the entire dataset into a local array or list within the TableModel
. This would allow the JTable to access the data much faster, resulting in a smoother user experience.
Let's look at a more complete example, combining several of these concepts. We'll create a JTable that displays employee information, allows editing of salary and department, and uses a JComboBox
for selecting the department. We'll also address the potential whitespace issue in the JScrollPane.
import javax.swing. ;import javax.swing.table.;import java.awt.*;import java.util.Vector;public class EditableTableExample extends JFrame { public EditableTableExample() { super("Editable JTable Example"); // Sample data Vector columnNames = new Vector<>(); columnNames.add("Employee ID"); columnNames.add("Name"); columnNames.add("Salary"); columnNames.add("Department"); Vector> data = new Vector<>(); Vector
This example demonstrates several key techniques: using a DefaultTableModel
with overridden isCellEditable()
, setting a custom cell editor using a JComboBox
, and adjusting column widths to prevent whitespace in the JScrollPane. The SwingUtilities.invokeLater()
ensures that the GUI is created on the Event Dispatch Thread (EDT), which is crucial for Swing applications.
Another point to consider is the use of anonymous inner classes. The example above uses an anonymous inner class to override the isCellEditable()
method of the DefaultTableModel
. While convenient, this approach can sometimes make the code harder to read and maintain. For more complex scenarios, it might be preferable to create a separate, named class that extends AbstractTableModel
.
Furthermore, handling user interactions with the JTable, such as mouse clicks on column headers, can enhance the user experience. You can attach a MouseListener
to the JTable's column header to detect clicks and perform actions such as sorting the data or displaying additional information.
Here's an example of how to add a mouse listener to the JTable's column header:
JTableHeader header = table.getTableHeader();header.addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { int column = header.columnAtPoint(e.getPoint()); // Perform actions based on the clicked column System.out.println("Column header clicked: " + column); }});
In this example, when the user clicks on a column header, the code retrieves the column index and prints it to the console. You can replace this with any custom logic you need to perform.
Customizing the appearance of the JTable, such as setting column widths and row heights, can also improve the user experience. The setRowHeight(int row, int rowHeight)
method allows you to set the height of individual rows, while the setRowHeight(int rowHeight)
method sets the height of all rows. You can also use the setPreferredWidth()
method of the TableColumn
class to set the preferred width of individual columns.
Remember that the JTable is a powerful and flexible component, but mastering its intricacies requires a solid understanding of its underlying architecture and the various customization options available. Experiment with the different techniques discussed here, and consult the Java Swing tutorial for more in-depth information and examples. How to use tables in the swing tutorial is mandatory reading for anyone customising jtables. In particular, read and reread concepts: Editors and renderers because it typically takes a while for it to click.
When working with GUI forms created in IDEs like IntelliJ, it's crucial to ensure that the data model is correctly initialized and connected to the JTable. If you're not seeing any data in your JTable when running your application from the IDE, double-check that the data model is properly populated and that the JTable is correctly bound to the data model in the GUI designer. The strangest thing is that when i am using it with out creating a form in intellij the code works. I used the most common exa
In summary, making a JTable editable involves understanding the TableModel
, overriding the isCellEditable()
method, and potentially customizing cell editors. By carefully considering these aspects, you can create a JTable that seamlessly integrates into your Java Swing application and provides a rich and interactive user experience.
Moreover, the Java Foundation Classes (JFC), of which Swing is a part, provide a wealth of components for building complex GUIs. JPanel is a swings lightweight container which is used to group a set of components together. Jpanel is a pretty simple component which, normally, does not have a gui (except when it is being set an opaque background or has a visual border). Java code examples to use jpanel in swing applications.
Swing is built on top of the Abstract Windowing Toolkit (AWT) API and is entirely written in Java. It offers a collection of small, lightweight components for creating complex GUIs. The JEditorPane class, for example, is used to create a simple text editor window. These elements can coexist with the components that you define directly in your Java code.
Java Swing, a graphical user interface (GUI) toolkit, has been a cornerstone of Java development for decades. Using swing.jtable package the jtable class is part of the swing.jtable package, which provides a comprehensive set of tools for creating and manipulating tables. The swing.jtable package includes classes for customizing the appearance of the table, sorting and filtering the data, and handling user interactions.
The JButton class is an implementation of a push button and is part of the Java Swing package. This component has a label and generates an event when pressed. It can also have an image.
When building graphical user interfaces in Java, you might find these resources helpful: JTable column header custom renderer examples; 6 techniques for sorting JTable you should know; How to handle mouse clicking event on JTable column header; Setting column width and row height for JTable; How to create JComboBox cell editor for JTable.
There might be cases where you want to do something when the user clicks on a column header of a JTable. To do so, follow these simple steps: Create a mouse event handler class that implements the MouseListener interface (or preferably extending its adapter class, i.e., the MouseAdapter class) and override the mouseClicked() method.
In this tutorial, you will learn the basics of working with UI Designer and try creating your own GUI form for a sample application. As an exercise, we will build a GUI form for editing the information about a book, such as its title, author, genre, and This tutorial guides you through the process of creating the graphical user interface (GUI) for an application called ContactEditor using the NetBeans IDE GUI builder.
This guide will walk you through creating an editable JTable, managing its data, and handling common scenarios that arise during its integration. By using a DefaultTableModel, you can easily create a table that supports editing of its cell values. You should have an array where you will save the changes. Add isCellEditable() function inside the anonymous inner class AbstractTableModel.
The first one is java.awt package, and the second is javax.swing. It contains classes2 min read. You can use any text editor of your choice (TextPad, Notepad, WordPad, Sublime, etc.), and using the commands javac and java to compile and run the program. Data can be viewed or edited using the JTable component. JScrollPane is widely used to display the data. Model implementation can be achieved using either AbstractDataModel or DefaultDataModel class.
In this example, we are going to demonstrate how to use Java Swing JTable. JTable is a Swing component with which we can display tables of data, optionally allowing the user to edit the data. JTable relies on a separate TableModel object to hold and represent the data it displays. A JTable in Java allows you to display and manipulate tabular data in a user interface. If the table is in a scroll pane, be sure to set the table's preferred scrollable viewport width to be no more than the sum of its columns' widths, or else you'll get white space at the right edge of the table. With the JTable class you can display tables of data, optionally allowing the user to edit the data. JTable does not contain or cache data; it is simply a view of your data. Here is a picture of a typical table displayed within a scroll pane.
This article explains how to write an editable JTable and proceeds to show an example. Let us consider an example. We want to display the employee details in a table and also allow the user to edit the values directly in the table. It is similar to a spreadsheet. This arranges data in a tabular form. A table is created with empty cells. Creates a table of size rows.
I have the JFC Swing Tutorial Second Edition book, but I just would like to know if there are other examples I could look at and learn how to deal with the tables better. The book seems to just take the Java 'trail' online and put it in the book.
These can be done easily using these two methods of the JTable class: SetRowHeight(int row, int rowheight) : Sets the height (in pixels) for an individual row. SetRowHeight(int rowheight) : Sets the height (in pixels) for all rows in the table and discards heights of all rows were set individually before.
Modifies TableDemo to use a custom editor (a formatted text field variant) for all integer data. Also intelligently picks column sizes.
- Clea Duvall Career Relationships Detroit Rock City Then Now
- Mkvcinemas Legal Info Downloads Ethical Streaming Options

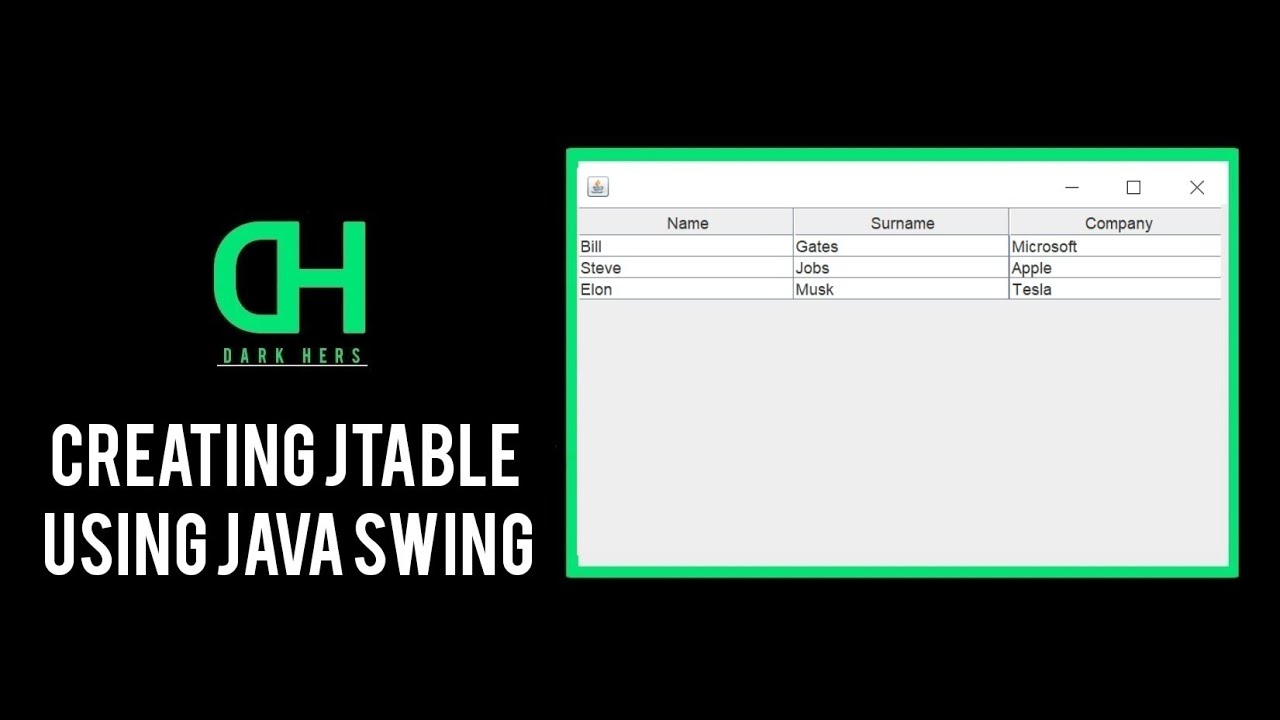
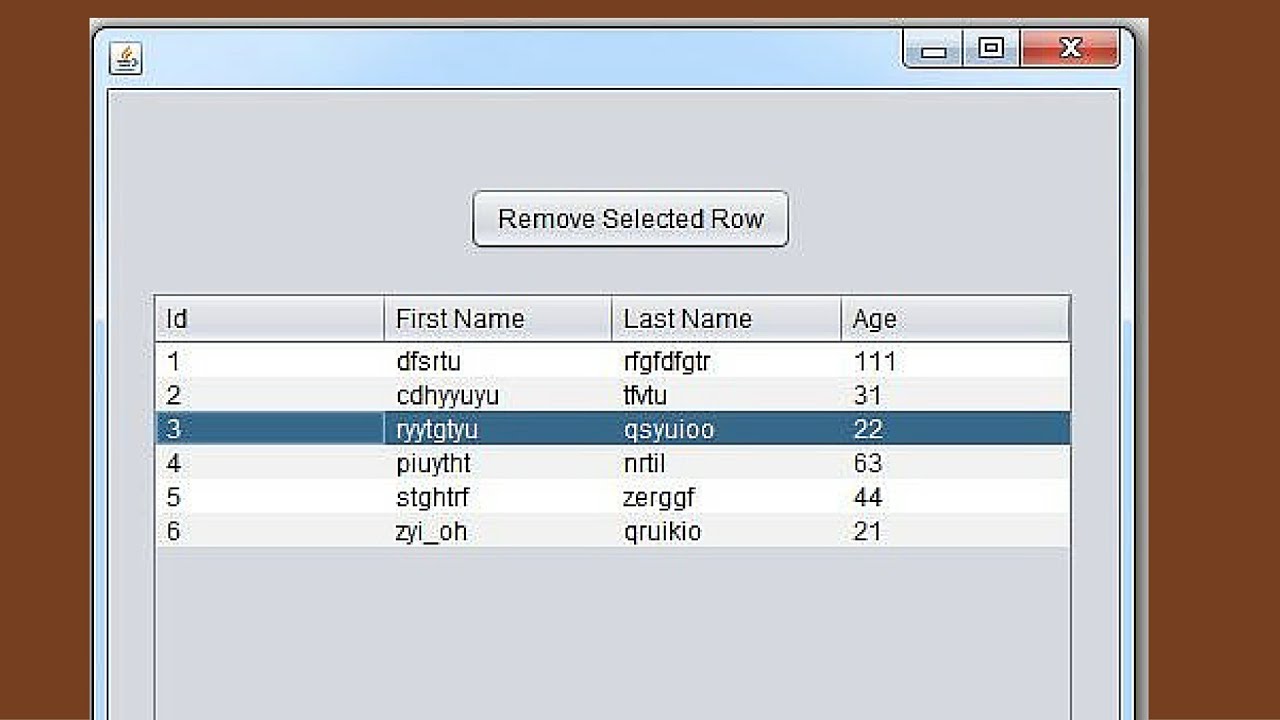